Key Features
Completion Certificate
Internship
Internship Certificate
7 Days Refund Policy
Expert Instructors
One-to-One Session
What Will You Learn?
Accelerate your learning journey with our comprehensive course designed to equip you with essential skills and practical knowledge in Full Stack Web Development with Django and React.
- Django Basics
- RESTful API Development with Django Rest Framework
- Frontend Development with React
- State Management with Redux
- Integrating React with Django Backend
- Authentication and Authorization
- Building and Deploying Full Stack Applications
Requirements
Before getting started with this course, it's beneficial to have the following:
- Laptop with good internet
- Passionate about learning web development
- No prior experience needed
- Willing to dedicate time
- Curious about building modern web applications
Course Completion
Yes
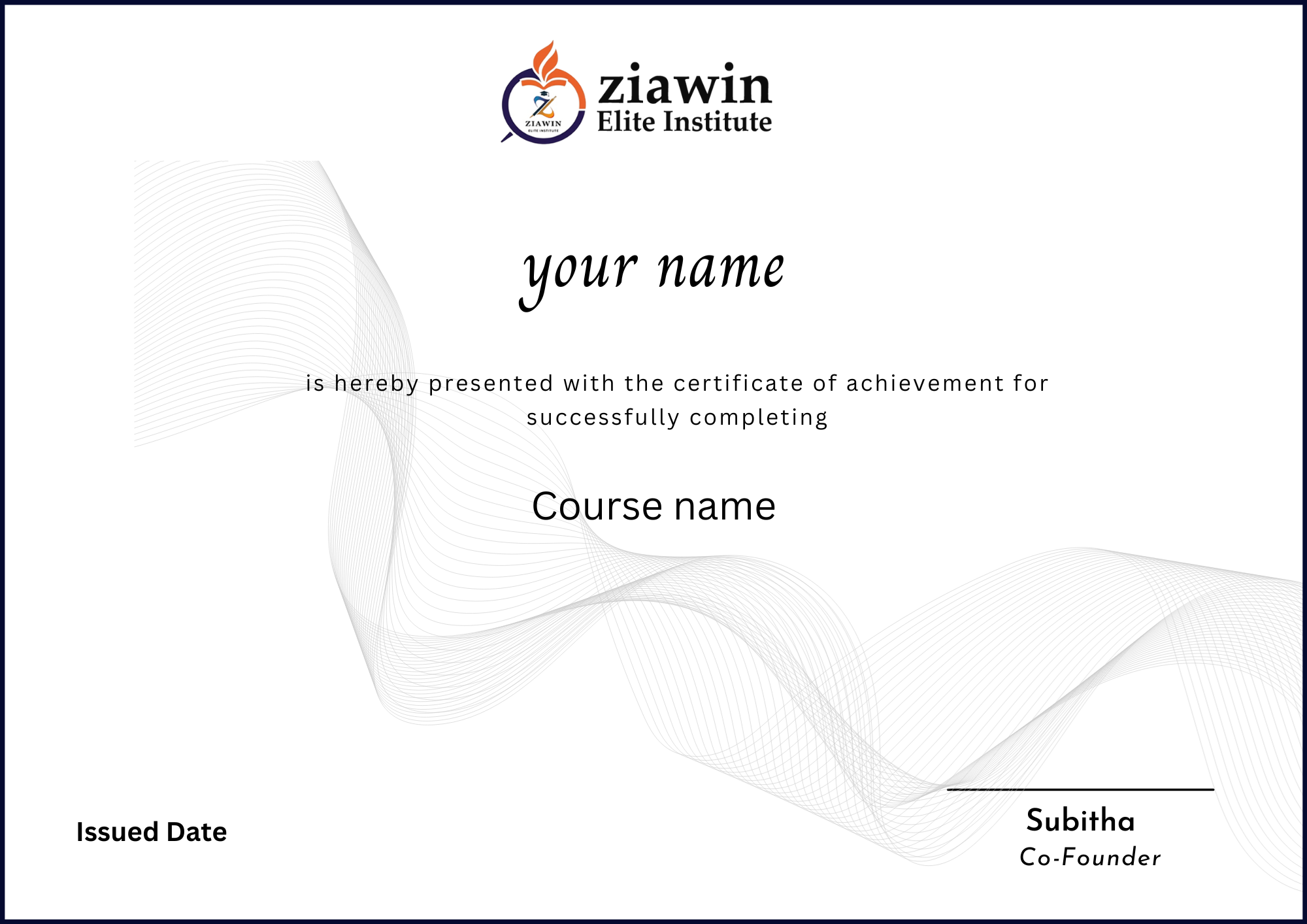
Curriculum
- Definition and Overview of the Web
- Understanding the Internet vs. the Web
- Components of the Web (Web Servers, Web Browsers, and Web Pages)
- The Role of HTTP and HTTPS
- How Websites and Web Applications Work
- Understanding Web Protocols (TCP/IP, DNS, etc.)
- The Evolution of the Web (Web 1.0, Web 2.0, Web 3.0)
- HTML Level Two Introduction
- HTML Level Two - Part One - Tables
- HTML Level Two - Part Two - Tables Quiz
- HTML Level Two - Part Three - Table Quiz Solutions
- HTML Level Two - Part Four - Forms Basics
- HTML Level Two - Part Five - Forms and Labels
- HTML Level Two - Part Six - Forms and Selections
- HTML Level Two - Assessment
- HTML Level Two - Assessment Solutions
- CSS Level One Part Three
- CSS Level One Part Four
- CSS Level One Part Five
- CSS Level One Assessment
- CSS Level One Assessment Solutions
- CSS Level Two
- CSS Level Two Introduction
- CSS Level Two - Part One - Fonts
- CSS Level Two - Part One (Fonts) Continued
- CSS Level Two - Part Two - Box Model
- CSS Level Two Spectrum Project Overview
- CSS Level Two Spectrum Project Solutions
- JS Level One Introduction
- JS Level One - Part One - Basics
- JS Level One - Part Two - Connecting Javascript
- JS Level One - Part Three - Exercise
- JS Level One - Part Three - Solutions
- JS Level One - Part Four - Operators
- JS Level One - Part Five - Control Flow
- JS Level One - Part Six - While Loops
- JS Level One - Part Seven - For Loops
- JS Level One Part Eight - Loop Exercises
- JS Level One - Part Eight - Solutions
- JS Level One - Project Overview
- JS Level One - Part Nine - Project Solutions
- Introduction to JavaScript - Level Two
- Functions in JavaScript - Part One
- Exercises on JavaScript Functions
- Solutions to Function Exercises
- Understanding JavaScript Arrays
- Exploring Array Exercises - Part One
- Solutions to Array Exercises
- Working with JavaScript Objects
- Deep Dive into Objects in JavaScript
- Object Exercises in JavaScript
- Solutions to Object Exercises
- Getting Started with Python Level One
- Python Setup and Installation
- Working with Numbers in Python - Part One
- Introduction to Strings in Python - Part Two
- Exploring Lists in Python - Part Three
- Understanding Dictionaries in Python - Part Four
- Working with Tuples, Sets, and Booleans - Part Five
- Overview of Python Exercises - Part Six
- Solutions for Python Exercises - Part Six
- Control Flow in Python - Part Seven
- Introduction to Functions in Python - Part Eight
- Function Exercises Overview - Part Nine
- Function Exercises Solutions
- Overview of Simple Game Project in Python - Part Ten
- Solutions for Simple Game Project - Part Ten
- Understanding Scope in Python
- Introduction to Object-Oriented Programming - Part One
- Exploring Object-Oriented Programming - Part Two
- Advanced Object-Oriented Programming - Part Three
- OOP Project Overview
- OOP Project Solutions
- Handling Errors and Exceptions in Python
- Regular Expressions in Python
- Working with Python Modules and Packages
- Introduction to Python Decorators
- Understanding the Name and Main Functionality
- Introduction to Social Media Clone Project
- Social Clone Part One
- Social Clone Part Two
- Social Clone Part Three
- Social Clone Part Four
- Social Clone Part Five
- Social Clone Part Six
- Social Clone Part Seven
- Social Clone Part Eight
- Social Clone Part Nine
- Social Clone Part Ten
- Social Clone Part Eleven
- Social Clone Part Twelve
- Social Clone Final Part
- Setting up the project structure
- Creating models for products, users, and orders
- Implementing product listing and search functionality
- User authentication and registration
- Shopping cart functionality
- Order management and checkout process
- Implementing payment gateway
- Order history and user dashboard
- Admin panel setup for managing products and orders
- Setting up project structure
- Creating models for tasks, categories, and users
- User authentication and registration
- Task creation, editing, and deletion
- Categorizing tasks and filtering by status
- Setting task due dates and notifications
- User dashboard with task progress overview
- Admin panel for task management
- Task collaboration (assigning tasks to users)
- Setting up the chat application structure
- User authentication and real-time user presence
- Implementing one-to-one and group chat functionality
- Setting up WebSockets for real-time communication
- Storing messages and user details in the database
- Sending notifications for new messages
- Chat interface and user experience (UI/UX)
- Admin panel for monitoring chats and users
- Securing chat data and privacy
- Setting up project structure
- Designing homepage with introduction and portfolio items
- Creating a contact form and email integration
- Showcasing projects with images, descriptions, and links
- Adding a skills and experience section
- Implementing a blog or articles section
- Making the website responsive for mobile devices
- SEO optimization for better visibility
- Deploying the website
- What is Debug Toolbar in Django?
- Installing and configuring Debug Toolbar
- Understanding Debug Toolbar panels
- Analyzing SQL queries, cache, and request/response details
- Using Debug Toolbar to debug template rendering
- Debugging request lifecycle and middleware
- Optimizing queries and improving performance
- Best practices for using Debug Toolbar in development
- Overview of Django Admin interface
- Customizing the Django Admin interface
- Creating models and registering them in Admin
- Adding filters, search, and ordering functionality
- Managing users, groups, and permissions in Admin
- Admin form customization and inline models
- Creating custom actions and buttons in Admin
- Setting up role-based access for Admin users
- What is DRF and why use it?
- Installing and setting up DRF in Django project
- Creating APIs using serializers and views
- Setting up URL routing for APIs
- Authentication and permissions in DRF
- Implementing CRUD operations for models via API
- Using pagination, filtering, and ordering in DRF APIs
- Setting up DRF for complex data models (relationships)
- Testing and documentation with DRF
- Introduction to React and its ecosystem
- Setting up React with Create React App
- Understanding React components and JSX
- State and props in React
- Component lifecycle methods
- Event handling in React
- Conditional rendering and loops in React
- Form handling in React
- React Router for navigation between pages
- React Project (Integrating with Django Back-End)
- Setting up Django backend with React frontend
- Exposing APIs in Django for React to consume
- Using Axios or Fetch to make HTTP requests from React
- Handling form submissions and validations in React
- Managing state in React using Context API or Redux
- Authentication with JWT tokens
- Error handling in React components
- Handling responses and updating UI dynamically
- Deploying Django and React together
- React Deployment
- Preparing the project for deployment
- Setting up React for production build
- Deploying React app to platforms like Netlify, Vercel, or Firebase
- Configuring environment variables for production
- Setting up the production environment in Django
- Configuring static files and media for deployment
- Deploying the combined Django + React app
- Debugging and monitoring deployed applications
- Post-deployment steps (e.g., database backups, scaling)
- Common interview questions for Django, React, and Full Stack development
- How to present your projects in interviews
- Coding challenges and problem-solving techniques
- Best practices for technical interviews
- Preparing for behavioral and HR interviews
- Mock interview sessions and tips
- Reviewing key concepts in Python, Django, React, and DRF
- Interview follow-up best practices
- Building a strong portfolio and resume
- Resume Discussions
- How to create an impressive developer resume
- Highlighting relevant skills and technologies
- Structuring your portfolio and project descriptions
- Tailoring your resume for specific job roles
- Showcasing your achievements and contributions
- How to write an effective cover letter
- Discussing your projects and experiences during interviews
- Key skills to mention for a web developer role
- Avoiding common mistakes in resumes